Building fast can sometimes lead to a codebase that’s as organized as a teenager’s bedroom. At Noxus, our adventures with React Flow taught us that a solid state management strategy is like having a clean desk—everything just works smoothly.
The Challenge: Dynamic Node State in React Flow
When creating our editor, we faced a burning question: How can we make our outside state and methods available to the nodes themselves? Most flow-based applications we saw had little to no sharing between the nodes and the state that surrounds the editor. However, this was not our case.
While the React Flow documentation promised to have your back, it turns out it only handles static information attached to the nodes. For us—with a bunch of moving parts—this approach was about as useful as a screen door on a submarine. In their defence, they did suggest using a 3rd party state management tool - but in our early days this felt like a huge overhead to add. You know what they say about hindsight…
The First Approach: Embracing Context
Our initial solution was to loosely trust React’s Context. We leveraged React’s useContext
hook to create an EditorContext
, which all nodes happily consumed. This allowed us to share our state and methods across the editor. Although we were aware about some performance issues with Context, it was just the simpler way to get the ball rolling.
const EditorContext = React.createContext();
function Editor({ children, state, methods }) {
const addNewDynamicOutput = useCallback(() => {
// Do a bunch of stuff here
// including manipulating the editor nodes
{...}
}, [nodes]);
return (
<EditorContext.Provider value={{ addNewDynamicOutput }}>
<ReactFlow {...} />
</EditorContext.Provider>
);
}
function Node() {
const { addNewDynamicOutput } = useContext(EditorContext);
// Node-specific logic here...
return <div>{/* ... */}</div>;
}
As you can see, this created a very easy way for us to loosely make anything we wanted available to the nodes. However, you might already be able to see what issues this caused.
The Hidden Performance Pitfall: When "Fast" Feels Like "Buffering..."
Using EditorContext
solved our state-sharing woes—until it didn’t. We soon encountered performance issues that made our development experience feel like watching a YouTube video in 144p. Sure, production was “ignoreable” (looking at you, React Strict Mode 👀), but we did start noticing things grew noticeable slower as the customer’s workflows got bigger and bigger.
Digging Deeper: What in the World Was Causing the Sluggishness?
We rolled up our sleeves and asked, “Why is everything so slow?” Turns out, our reducer methods and external UI library integrations were causing more re-renders than a cat video goes viral.
Enter Our New Best Friend: React DevTools’ Profiler
Time to call in the big guns: the React DevTools Profiler. I once read a tweet claiming every React developer should always have “highlight when a component re-renders” turned on. Sure, it might feel like overkill—like bringing a bazooka to a pillow fight—but trust us, the insights are worth it. Look at the gif below to see what could have been avoided if we had this enabled since the beginning…
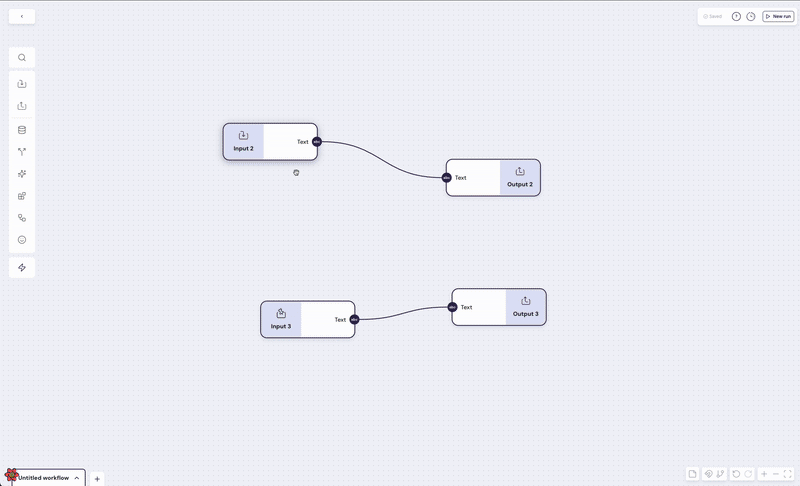
ℹ️ Pro tip: enable “what caused this update” checkbox on the React Profiler. It'll the analysis a tad slower, but the insights are very good.
Diagnosing the Culprits
After some sleuthing, we identified two main issues:
1- Reducer Methods with Unintended Dependencies
We defined our reducer methods using useCallback
, and by including the nodes array (which changes every time a node moves) in the dependency list, every time you dragged a node, it was like triggering a chain reaction of re-renders.
2- Chakra UI’s Popover are a bit sub-optimal by default
Our node picker menu used Chakra UI’s Popover
component with nested menus. With the default isLazy
set to false
(why???), popover’s content was always rendered—even when hidden—making our UI work harder than a caffeinated hamster.
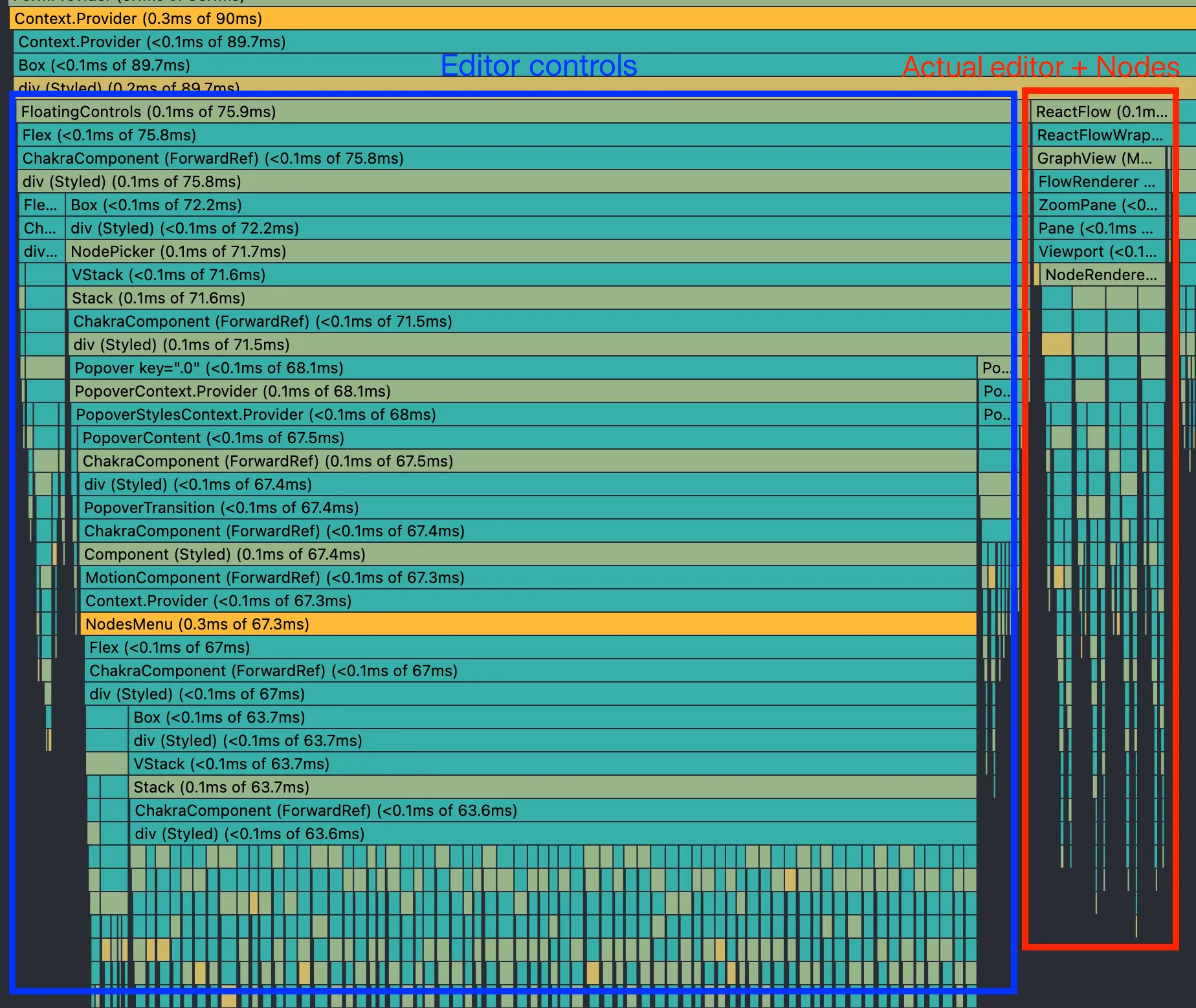
Two-Pronged Solutions: Quick Wins and the Real Deal
Quick Win: Enabling isLazy
on Popovers
Our first hack was simple: set isLazy
to true
on our popovers. This little tweak was like turning off the lights in a haunted house—it immediately reduced the number of elements rendered during re-renders.
While this was a lifesaver, it didn’t completely solve the performance lag when dealing with a zoo of nodes - we were aware this was not enough, it bought us some time.
Long-Term Solution: Proper state-management
For a real fix, we overhauled our state management. We moved all dynamic state to Jotai stores. There’s a world of state management tools for you to use, that would suit this use case. We went with Jotai because of it’s low boilerplate and ease of use.
This allowed us to:
- Keep state performance on point.
- Use Jotai’s atoms for state reducers, avoiding the recalculation nightmare caused by our previous useCallback dependency chain.
The result? On workflows with 50+ nodes, our editor went from a laggy 10fps to a smooth 60fps— Carlos Santana would be proud 🎸
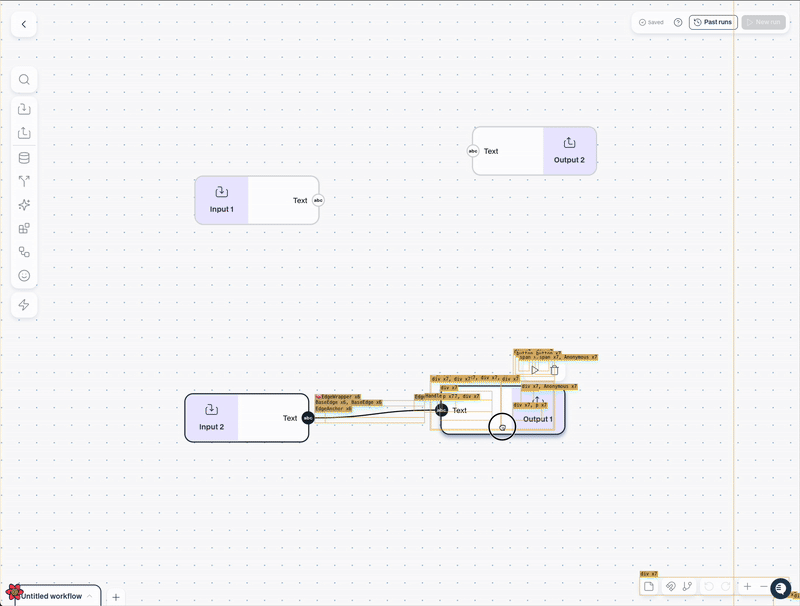
Join the Noxus Adventure!
At Noxus, we tackle complex challenges in AI and Software Engineering every day. If you’re passionate about solving problems, love a good meme, or just want to work on cutting-edge solutions, we’re hiring!
Thanks for reading! Got questions or suggestions to share? Drop a comment below or reach out to us directly. Happy coding, and may your state always be managed! ⚖️